Python Tuples: A Faster and Secure Data Structure
- Pankaj Maheshwari
- Jan 25, 2023
- 3 min read
Updated: Jul 3, 2023
A tuple is a data type in Python that is similar to a list, but it is immutable, which means that once you create a tuple, you cannot change the elements it contains by adding, removing, or modifying elements. This makes tuples more efficient and easier to work with in certain cases because you don't have to worry about the elements being modified unexpectedly.
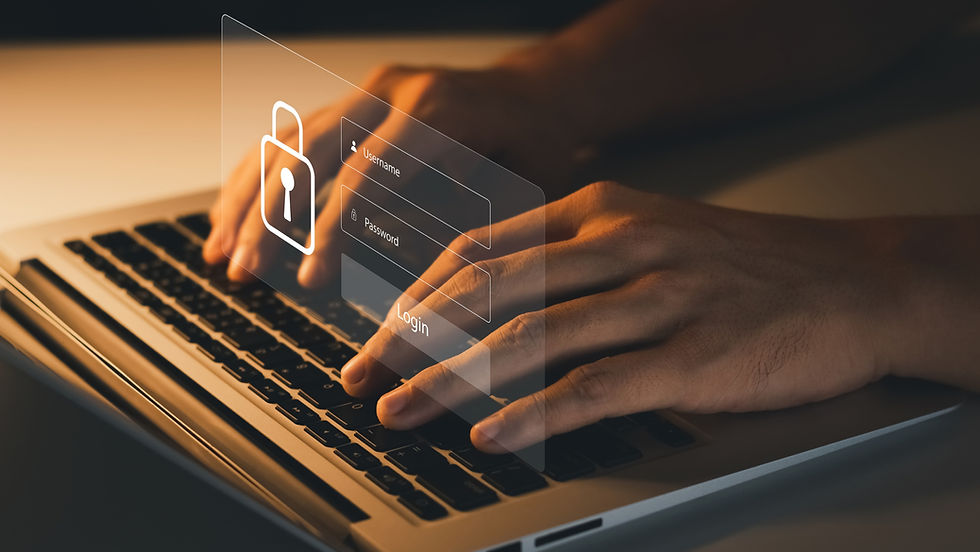
To create a tuple, you use parentheses () and separate the items with commas.
test_tuple = ('John', 'Lucy', 'Mike', 'Harry')
Basic Functions and Methods for Python Set
There are several built-in methods and functions that you can use to work with tuples.
len(): the len() function returns the length of a tuple, which is the number of elements it contains.
test_tuple = ('John', 'Lucy', 'Mike', 'Harry')
result = len(test_tuple)
print(result)
Output: 4
It is a very useful function for determining the size of an object, and it is often used in looping constructs to iterate over the elements of an object.
min() and max(): the min() and max() functions return the minimum and maximum values in a tuple, respectively. These functions work by comparing the elements of the tuple and returning the smallest or largest element based on their values.
test_tuple = (1, 3, 5, 7, 9)
result_1 = min(test_tuple)
result_2 = max(test_tuple)
print(result_1, result_2)
Output: 1 9
They are useful for quickly finding the minimum or maximum value in a collection of values.
Keep in mind that the min() and max() functions only work with values that can be compared using the < and > operators. If the tuple contains elements that cannot be compared in this way, the functions will raise a TypeError exception. For example, if the tuple contains both integers and strings, the functions will not work because you cannot compare integers and strings using the < and > operators.
count(): the count() method returns the number of times a specific value appears in a tuple.
test_tuple = ('John','Lucy','John','Mike','Harry','John','John')
result = test_tuple.count('John')
print(result)
Output: 4
It is a useful method for quickly finding out the number of occurrences of any value in a tuple.
Keep in mind that the count() method only counts the number of occurrences of an exact value. For example, if you have a tuple containing the string "apple" and you try to count the number of occurrences of the string "app", the count() method will return 0 because there are no occurrences of the string "app" in the tuple.
index(): the index() method returns the index of the first occurrence of a specific value in a tuple.
test_tuple = ('John', 'Lucy', 'Mike', 'Harry')
result = test_tuple.index('John')
print(result)
Output: 0
If the value is not found in the tuple, the method will raise a ValueError exception.
You can also use the index() method to find the index of a value within a specific range of indices in the tuple. To do this, you can pass a start and stop index as arguments to the index() method.
test_tuple = ('John', 'Lucy', 'Mike', 'John', 'Harry')
index = test_tuple.index('John', 2, 5)
print(index)
Output: 3
This will search for the first occurrence of the element 'John' in the tuple starting at index 2 and ending at index 5 (exclusive).
Benefits of Using Tuples in Python
Python tuples are an important data structure with benefits, including:
Once you create a tuple, you cannot change its contents by adding, removing, or modifying elements. This can be useful in cases where you want to ensure that the contents of an object are not modified by mistake.
Tuples are generally faster to access and iterate over than lists because they are implemented using a more efficient data structure. This can be important in cases where you need to perform a lot of operations on a large collection of data.
Unlike lists, tuples can be used as keys in dictionaries because they are immutable. This can be useful for creating dictionaries where the keys are tuples of values.
Comments