Understanding Variables in Python: Declaration, Assignment, and Naming Conventions
- Pankaj Maheshwari
- Jun 1
- 7 min read
Updated: Jun 17
In programming, a variable is a symbolic name that refers to a value stored in the computer’s memory. You can think of it as a label for data. By giving a descriptive name to some data, you can easily reuse and manipulate that value throughout your code. Variables are essential building blocks of programs, they let you store information (like numbers, text, etc.) and use it later, making your code flexible and dynamic. In short, a variable allows you to save a value with a name and then refer to that value by name as needed.
Assigning Values to Variables in Python
Creating a variable in Python is straightforward: you simply write a variable name, then the assignment operator =, and then the value you want to store. This automatically creates the variable and assigns it the given value; there’s no separate “declaration” step or type specification required.
stringVariable = "Hello World!"
integerVariable = 1234567890
In the above code, `pythonVariable` is a variable set to the string "Hello world!", and `integerVariable` is a variable set to the number 1234567890. Python creates these variables at the moment of assignment. Internally, Python will create an object to represent the value (like the number 1234567890) and make the variable name point to that object in memory. As a beginner, you can imagine that the value gets “stored” in the variable.
Python is a dynamically typed language, meaning you do not need to declare a variable’s type ahead of time; the type is inferred from whatever value you assign. In fact, Python variables don’t have a fixed type at all; the same variable can hold a value of one type now and a different type later.
You can first assign a number to a variable and then assign a string to the same variable:
pythonVariable = "Hello World!"
pythonVariable = 1234567890
In this example, Python initially treats `pythonVariable` as a string (when pythonVariable = "Hello World!") and later as an integer (when pythonVariable = 1234567890). This is allowed because Python variables are dynamically typed, meaning the same variable can hold different types of values as the program runs. You don’t ever need to write something like “str pythonVariable” or “int pythonVariable”, just assign the value directly, and Python will figure out the type from the value.
The assignment operator (=) is used to assign values to variables. It doesn’t represent equality (as in math) but rather the action of storing a value in a variable. For instance, x = 5 means “assign the value 5 to the name x.” Each assignment statement executes right-to-left: Python evaluates the expression on the right side and then binds the result to the variable name on the left side. If you write y = x, it will make y reference the same value that x currently holds (no new copy of the object is made). Variables are created when you first assign them a value, and you must assign a value before using a variable’s name in your code (otherwise, you’ll get a NameError because the name doesn’t exist yet).
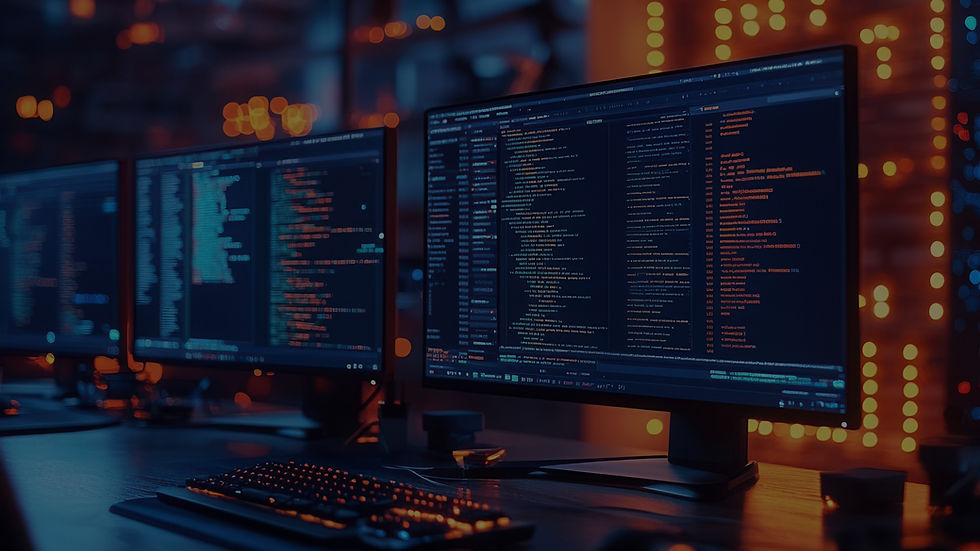
Examples of Variable Assignment
Let’s look at a few simple examples to illustrate different ways to assign variables:
Single Variable Assignment: You can assign a value to a single variable using =.
pythonVariable = "Hello World!"
print(pythonVariable)
Here, we created a single variable and assigned it the string "Hello World!". Using print(pythonVariable) will output the value stored in name (which is "Hello World!").
Variable Reassignment: You can change the value of an existing variable by assigning it a new value.
pythonVariable = "Hello World!"
print(pythonVariable)
pythonVariable = "Hello Universe!"
print(pythonVariable)
First, `pythonVariable` is set to "Hello World!". Later, we assign pythonVariable = "Hello Universe!", which updates the variable to hold a new value. The old value ("Hello World!") is no longer referenced by `pythonVariable`. You can even assign a value of a different type to the same variable, as shown earlier (e.g., assigning an integer to pythonVariable after storing a string). This flexibility is due to Python’s dynamic typing.
Multiple Variable Assignment (Chained Assignment): Python allows you to assign a value to multiple variables in one line.
a = b = c = "Hello World!"
print(a, b, c)
In this single line, `a`, `b`, and `c` are all assigned the value "Hello World!" simultaneously. After this line, `a`, `b`, and `c` all refer to the same value "Hello World!". (Internally, all three names point to one integer object with value "Hello World!".) If you later change one of them to a new value, the others remain at "Hello World!", since you then break the shared reference by reassigning one of the variables.
Multiple Variable Assignment with Different Values: Python also supports assigning different values to multiple variables in one statement. You do this by separating the variable names and values with commas.
a = b = c = 3, 6, 12
print(a, b, c)
In this case, `x` gets the value 3 (an integer), `y` gets 6 (an integer), and `z` gets 12 (an integer) all in one line. This is a convenient way to initialize several variables at once. The number of variables on the left should match the number of values on the right. This feature (also known as parallel/simultaneuous assignment or unpacking) makes code concise and easy to read.
Python Variable Naming Rules
When choosing names for your variables, you need to follow Python’s rules for valid identifiers (variable names). If a name breaks these rules, your code will not run. The basic naming rules in Python are:
Allowed characters: Variable names can contain letters (uppercase A-Z and lowercase a-z), digits (0-9), and the underscore. For instance, `pythonvar`, `pythonVar`, `pythonVar2`, and `pythonVar_2` are all valid names.
Variable Name Cannot Start with a Number: The first character of a variable name cannot be a number. For instance, `pythonVar2` is valid, but `2pythonVar` is not valid (it will cause a syntax error).
No Special Characters or Spaces in Varriable: Aside from underscores, you cannot use symbols like @, #, -, spaces, or other punctuation in variable names. For instance, `python-Var` is not a valid name (hyphens are not allowed).
Variable Names are Case-Sensitive: This means `pythonvar`, `pythonVar`, and `PYTHONVAR` are all seen as different, unrelated variables by Python. Be mindful that using different casing can confuse readers of your code, so avoid using the same word with different cases.
No Reserved Keywords: You cannot use Python’s reserved keywords as variable names. Keywords are the words that have special meaning in Python’s syntax (such as `if`, `else`, `for`, `while`, `class`, `True`, `False`, etc.). For instance, class = 10 is invalid because class is a keyword in Python. (If you accidentally choose a name that clashes with a keyword, you’ll get a syntax error. Simply pick a different name that isn’t a Python keyword.)
Best Practices for Naming Variables
Beyond the strict rules above, there are conventions and best practices that Python programmers follow to make code more readable and maintainable. Adopting these practices will help make your programs clearer both to yourself and others:
Use Descriptive Names: Choose a name that reflects the purpose of the variable or the data it holds. For example, use temperature instead of just `t`, or userName or user_name instead of `u`. A descriptive name makes code self-documenting. Avoid overly generic names like data or value that don’t convey meaning (except in trivial examples or loop indices).
Follow “snake_case” Convention: In Python, the standard style is to use lowercase letters for variable names, with words separated by underscores if needed. This is called snake_case. For example: `user_name` or `total_score`. Using underscores to separate words greatly improves readability compared to run-together names (username or totalscore). It allows you to quickly see the individual words, making the code clearer and more maintainable
Follow “camelCase” Convention: In some programming languages and coding styles (such as JavaScript or Java), it is common to use the camelCase convention for naming variables. In camelCase, the first word begins with a lowercase letter, and each subsequent word starts with an uppercase letter, without using underscores. For example, `userName` or `totalScore`. Using camelCase improves readability by visually separating words through capital letters rather than underscores. While Python’s official style guide (PEP 8) recommends using snake_case for variable names, you might still encounter camelCase in codebases influenced by other languages or frameworks. Therefore, it’s helpful to recognize and understand it.
As mentioned, Python is case-sensitive, so it’s best to use lowercase variable names consistently. Avoid using two names that differ only by case (like `user_name` and `userName`) as this can lead to confusion and errors. By convention, variable names generally start with a lowercase letter. (Uppercase names are typically used for constants by convention, or class names in camelCase – but as a beginner, you can remember that regular variables should be lowercase.)
Avoid Single-Character Names (except in simple cases): Single-letter variable names such as `x`, `i`, or `j` are fine in small contexts (like loop counters or mathematical formulas), but in most cases you should use more meaningful names. A name like `n` or `i` might be fine in a short loop, but for a variable that persists or is used in multiple places, `count` or `index` is clearer than `i`. Longer, descriptive names make the code easier to understand.
Avoid Confusing Abbreviations: In general, spell out words in your variable names for clarity (e.g., use document instead of doc, message instead of msg). Well-known abbreviations or conventions are an exception (for example, `url` is commonly understood to mean “Uniform Resource Locator”, so `url` is fine). The goal is to make sure someone else (or you, after some time) can read the code and immediately grasp what each variable represents.
Don’t Use Names that Shadow Built-Ins: This is a more advanced tip, but avoid naming your variables the same as built-in Python functions or types (like list, str, max, etc.). Using such names isn’t a syntax error, but it can override the built-in, leading to confusion or bugs. For a beginner, the main takeaway is to stick to unique, descriptive names that won’t conflict with Python’s own names.
By following these naming conventions and practices, your code will be clearer, more readable, and easier to maintain. Writing code is as much for humans to read as it is for the computer to execute, so choosing good variable names is an important habit to build early on.
Comments